So I created a binding of
FastNoise .
Thats how it looks in gideros:
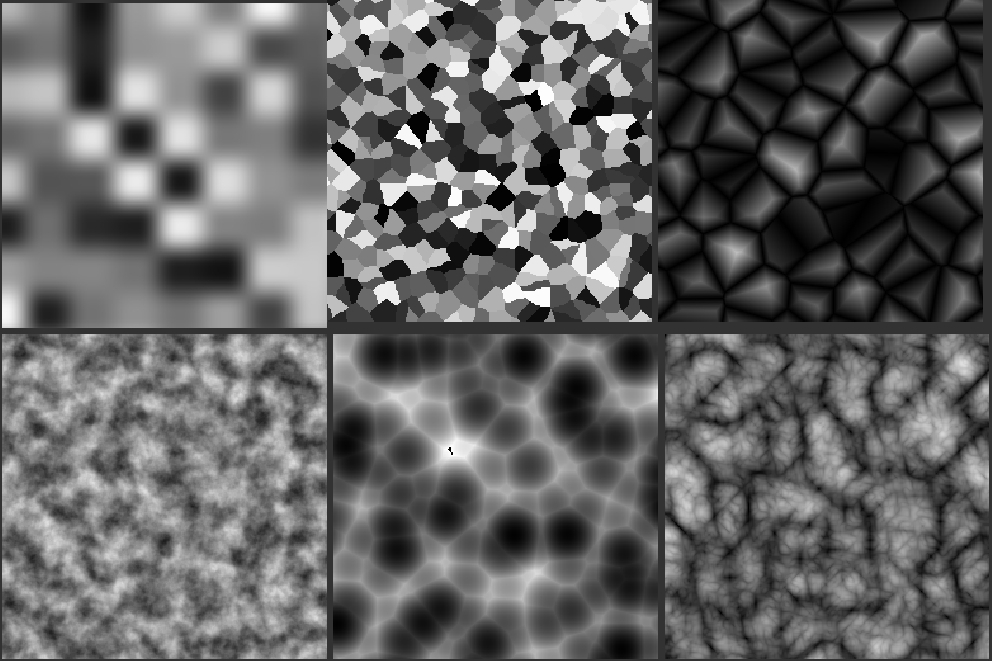
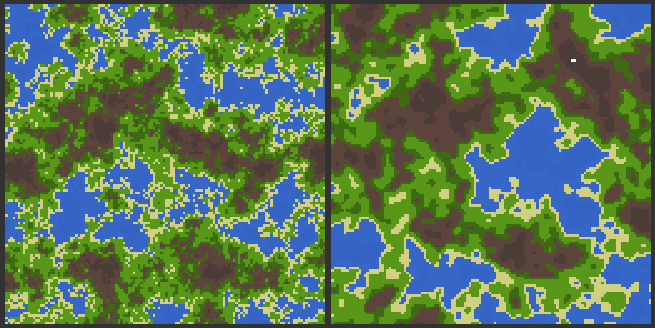
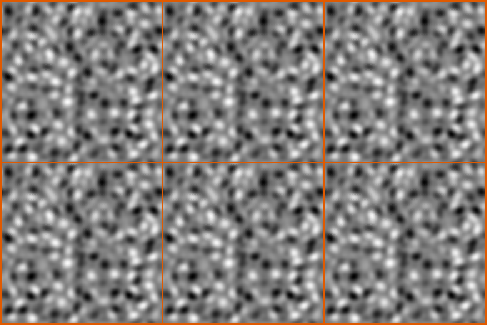
List of all methods:
Gideros only:
- reset() - reset noise parameters to default
- generateTexture(w, h, [filtering, zoff]) - returns grayscaled texture (no wrap support yet)
- generateTileableTexture(w, h, [filtering, zoff]) - returns grayscaled seamless texture (no wrap support yet)
- generateArray(n, [offset]) - returns a table with noise values (n - number of elements, offset - noise offset [optional])
Noise functions:
- noise([x, y, z]) - get 1D or 2D or 3D noise value (all parameters optional, defaults: 0)
- noise1D(x) - get 1D noise value
- noise2D(x, y) - get 1D noise value
- noise3D(x, y, z) - get 1D noise value
- whiteNoise4D(x, y, z, w) - get 4D white noise value (others availiable using methods above, just set noise type to Noise.WHITE_NOISE)
- whiteNoise1DInt(x) - get integer 1D white noise
- whiteNoise2DInt(x, y) - get integer 2D white noise
- whiteNoise3DInt(x, y, z) - get integer 3D white noise
- whiteNoise4DInt(x, y, z, w) - get integer 4D white noise
- simplex4D(x, y, z, w) - get 4D simplex noise
This methods work a bit differently compared to original.
- gradientPerturb1D(x) - returns modified x
- gradientPerturb2D(x, y) - returns modified x, y
- gradientPerturb3D(x, y, z) - returns modified x, y, z
- gradientPerturbFractal1D(x) - same as gradientPerturb1D
- gradientPerturbFractal2D(x, y) - same as gradientPerturb2D
- gradientPerturbFractal3D(x, y, z) - same as gradientPerturb3D
Noise options (see
https://github.com/Auburns/FastNoise/wiki):
- Noise:setNoiseType(noiseType): Default: Noise.SIMPLEX
Sets the type of noise returned by noise()
Possible noise types:
- Noise.VALUE
- Noise.VALUE_FRACTAL
- Noise.PERLIN
- Noise.PERLIN_FRACTAL
- Noise.SIMPLEX
- Noise.SIMPLEX_FRACTAL
- Noise.CELLULAR
- Noise.WHITE_NOISE
- Noise.CUBIC
- Noise.CUBIC_FRACTAL
- Noise:getNoiseType() returns noise type (number [VALUE = 0, VALUE_FRACTAL = 1, PERLIN = 2, PERLIN_FRACTAL = 3, SIMPLEX = 4, SIMPLEX_FRACTAL = 5, CELLULAR = 6, WHITE_NOISE = 7, CUBIC = 8, CUBIC_FRACTAL = 9])
- Noise:setFractalOctaves(n): Default: 3
The amount of noise layers used to create the fractal
Used in all fractal noise generation
- Noise:getFractalOctaves() returns number of fractal octaves
- Noise:setInterp(interpolation): Default: Noise.QUINTIC
Changes the interpolation method used to smooth between noise values
Used in Value and Perlin Noise
Possible interpolation methods (lowest to highest quality):
- Noise.LINEAR
- Noise.HERMITE
- Noise.QUINTIC
- Noise:getInterp() returns interpolation type (number [LINEAR = 0, HERMITE = 1, QUINTIC = 2])
- Noise:setSeed(seed) Default: 1337
Using different seeds will cause the noise output to change
Used in all noise generation
- Noise:getSeed() returns generator's seed
- Noise:setFrequency(frequency): Default: 0.01
Affects how coarse the noise output is
Used in all noise generation except White Noise
- Noise:getFrequency() returns noise frequency
- Noise:setFractalLacunarity(lacunarity): Default: 2.0
The frequency multiplier between each octave
Used in all fractal noise generation
- Noise:getFractalLacunarity() returns noise lacunarity
- Noise:setFractalGain(gain): Default: 0.5
The relative strength of noise from each layer when compared to the last
Used in all fractal noise generation
- Noise:getFractalGain() return noise gain
- Noise:setFractalType(noiseType): Default: Noise.FBM
Used in all fractal noise generation
Possible fractal types:
- Noise.FBM
- Noise.BILLOW
- Noise.RIGID_MULTI
- Noise:getFractalType() returns noise type (number [FBM = 0, BILLOW = 1, RIGID_MULTI = 2])
- Noise:setCellularDistanceFunction(distanceFunctionType): Default: Noise.EUCLIDEAN
The distance function used to calculate the cell for a given point
Possible distance functions:
- Noise.EUCLIDEAN
- Noise.MANHATTAN
- Noise.NATURAL
Natural is a blend of Euclidean and Manhattan to give curved cell boundaries
- Noise:getCellularDistanceFunction() returns distance function type (number [EUCLIDEAN = 0, MANHATTAN = 1, NATURAL = 2])
- Noise:setCellularReturnType(returnType): Default: Noise.CELL_VALUE
What value does the cellular function return from its calculations
Possible return types:
- Noise.CELL_VALUE
- Noise.NOISE_LOOKUP
- Noise.DISTANCE
- Noise.DISTANCE_2
- Noise.DISTANCE_2_ADD
- Noise.DISTANCE_2_SUB
- Noise.DISTANCE_2_MUL
- Noise.DISTANCE_2_DIV
Only CellValue is bounded between -1.0 and 1.0, all distance return types have a minimum of 0 and varying maximums
NoiseLookup requires another Noise object be set with setCellularNoiseLookup() to function
- Noise:getCellularReturnType() returns cellular return type (number [CELL_VALUE = 0, NOISE_LOOKUP = 1, DISTANCE = 2, DISTANCE_2 = 3, DISTANCE_2_ADD = 4, DISTANCE_2_SUB = 5, DISTANCE_2_MUL = 6, DISTANCE_2_DIV = 7])
- Noise:setCellularNoiseLookup(Noise): Noise used to calculate a cell value if cellular return type is NoiseLookup
The lookup value is acquired through GetNoise() so ensure you SetNoiseType() on the noise lookup, value, perlin or simplex is recommended.
- Noise:getCellularNoiseLookup() returns Noise object that was set by setCellularNoiseLookup() function
- Noise:setCellularDistance2Indices(index0, index1): Default: 0, 1
Sets the 2 distance indicies used for distance2 return types
Note: index0 should be lower than index1
Both indicies must be >= 0, index1 must be < 4
- Noise:setCellularJitter(jitter): Default: 0.45
Sets the maximum distance a cellular point can move from its grid position
Note: Setting this high will make cellular grid artifacts more common
- Noise:getCellularJitter() returns cellular jitter value
- Noise:setGradientPerturbAmp(amp): Default: 1.0
Sets the maximum perturb distance from original location when using gradientPerturb()
- Noise:getGradientPerturbAmp() returns gradient perturb amplitude.
Comments
Likes: rrraptor
Likes: rrraptor
P.S. Playing around
Likes: Apollo14, MoKaLux
Oh, does your library make fast 1D noise also? That's very handy for 2D games
From readme:
@hgy29 well, I've spent all day trying to get VS to work, but no success
But what now?)
Likes: MoKaLux
Likes: MoKaLux
But, I cant open it?
EDIT:
Never mind! I've successfully compiled sample plugin, but my code from 1st post still does not work! Same errors...
But clipper plugin compiles correctly. wtf is going on here...?
LIBS += -L"../../../Sdk/lib/desktop" -llua
And I thought that it is the same as in clipper.pro, but it turns out that I have copied bitop libs path))))
so I guess I just need to add -lgid to make it work
Back to VS
There is a tutorial of how to build gideros from surces but seems like I will only get dll's. Any tips?)
P.S. If you planning to release new version of gideros during this week, please let me know, I also want to add this binding with this release
EDIT:
QT build works
Likes: Apollo14, hgy29, SinisterSoft, MoKaLux
@antix can you test 1D noise?)
I've attached a plugin. Put it into "Plugins" folder inside you Gideros installation folder.
WORKS ONLY FOR WINDOWS
API can be found here: https://github.com/Auburns/FastNoise/wikiBut it is a little bit different.
For noise functions use FNoise.Noise1D(x) or FNoise.Noise2D(x, y) or FNoise.Noise3D(x, y, z)
For interpolation method (SetInterp) values are:
Binding for 4D WhiteNoise (WhiteNoiseInt) and 4D SimplexNoise is not implemented (sorry, I forgot about these).
Quick "HowTo":
But you may face various issues if you want to use VS, in particular with C++: different compilers may organize C++ internal machinery differently, so interopertaing C++ code from different compilers may not work as expected. For steam the API was pretty much 'C' only between gideros and the plugin.
Anyway, sincere congratulations for your first plugin!
Likes: MoKaLux
Likes: MoKaLux
- being able to generate a full array of noise values in a single call
- being able to make a texture from them, either greyscale or color if supplying a gradient pattern
I've found this in Box2D plugin:
For args, the data is a string containing R,G,B,A values (one byte per channel) for each pixel, so a 4xWidthxHeight char array in C, then you'll just need to:
Likes: rrraptor, SinisterSoft
Likes: rrraptor
In lua, its easy
Hmm...why gideros Texture/RenderTarget dont have some sort of "loadPixels" function... It would be useful for realtime texture generation. Instead of creating new texture every time, we create pixel data for existing one.
Likes: rrraptor
The interface looks like that:
filtering (boolean, default - false) indicate that the texture should be filtered [optional]
noiseScale (number, default - 1) noise scale factor [optional]
noiseZOffset (number, default - 0) noise z scroll [optional]
1024x1024 texture generates in 0.3 seconds (i7-2600k).
In realtime generating 256x256 texture EVERY frame drops fps to 40+ (classic Perlin noise)
256x256 using simplex noise gives stable 60 fps
Likes: MoKaLux
A bit of profiling:
Number of tests: 10000000
test1 6.0592579999998
test2 1.9603821000005
Number of tests: 1
test3 0.041751500000146
test4 0.13199930000019
test5 0.49677059999999
test6 1.9523522999998
Test1: generate noise array from lua (len - 1000000)
Test2: generate noise array from C (same len)
Test3: generate single 128x128 texture
Test4: generate single 256x256 texture
Test5: generate single 512x512 texture
Test6: generate single 1024x1024 texture
Likes: antix, MoKaLux
https://play.google.com/store/apps/developer?id=razorback456
мій блог по гідерос https://simartinfo.blogspot.com
Слава Україні!
Likes: oleg, MoKaLux
https://github.com/MultiPain/Gideros_FastNoise_binding
I've uploaded sources.
Im absolutely not sure about this original methods:
Also, 1D noise still broken, idk how to fix it. I think I'll just add Perlin and simplex noises for it, without fractals and other stuff.