Was tinkering and had some advice from some friends about alternative ways to do bloom and I realised that it could be done without using a custom shader at all - just standard Gideros commands, the problem was that it over-bloomed and needed fading, so I used the setColorTransform to fade and keep the bloom under control. The result is kinda pretty...
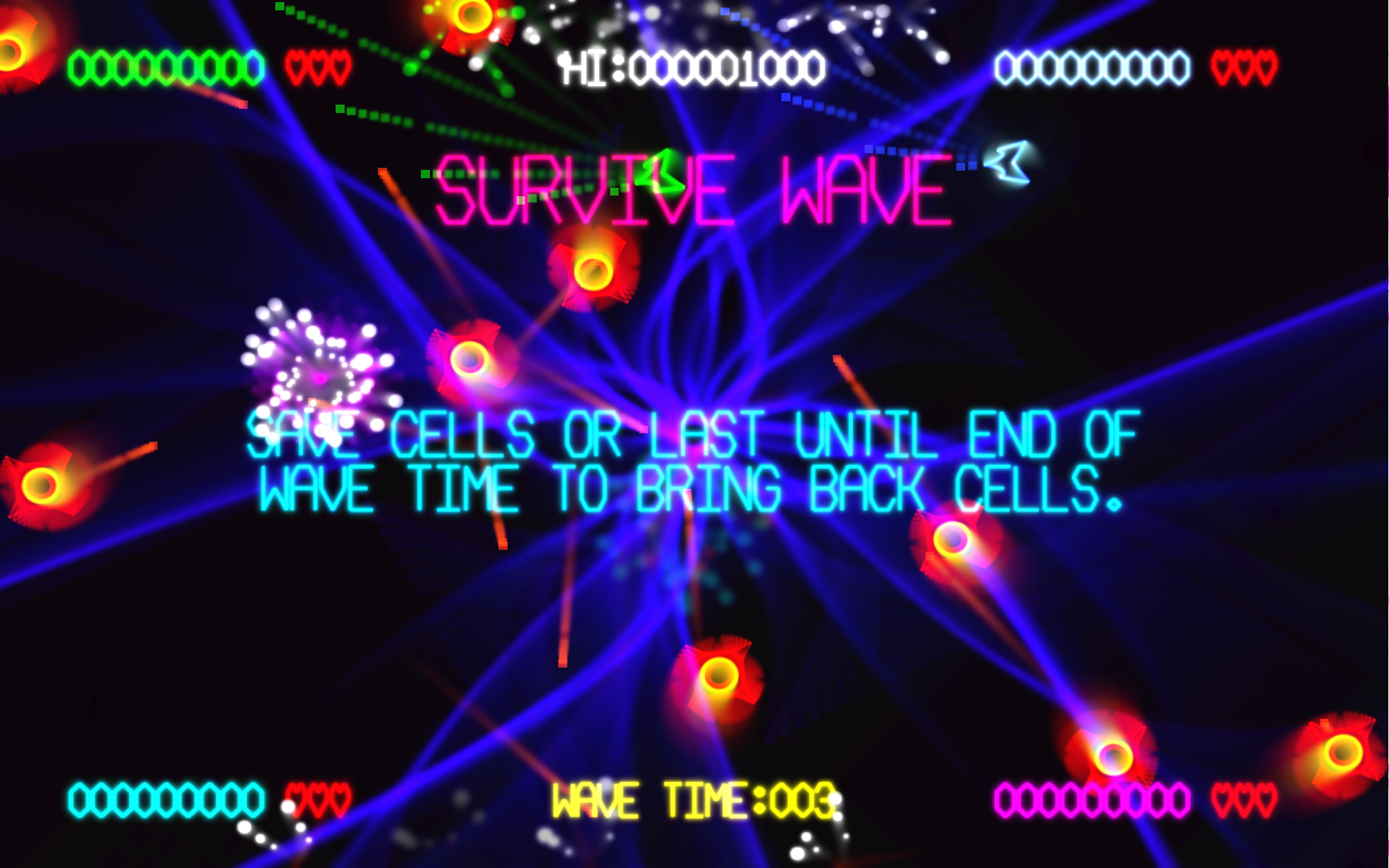
First you need to setup the screen
oddEven=0
local width=application:getContentWidth()
local height=application:getContentHeight()
local screenSizeX,screenSizeY=application:getDeviceHeight(),application:getDeviceWidth()
bloomScale=2
screenTexture={}
screenDraw={}
bloomCapture={}
oldScreen={}
for loop=1,2 do
screenTexture[loop]=RenderTarget.new(screenSizeX,screenSizeY,true)
screenTexture[loop]:clear(0,1)
screenDraw[loop]=Bitmap.new(screenTexture[loop])
screenDraw[loop]:setScale(width/screenSizeX,height/screenSizeY)
screenDraw[loop]:setVisible(false)
stage:addChild(screenDraw[loop])
end
bloomCapture[1]=Bitmap.new(screenTexture[2])
bloomCapture[2]=Bitmap.new(screenTexture[1])
bloomCapture[1]:setScale(1/bloomScale)
bloomCapture[2]:setScale(1/bloomScale)
print(screenSizeX,screenSizeY)
bloomScreen=RenderTarget.new(screenSizeX/bloomScale,screenSizeY/bloomScale,true)
bloomSprite=Bitmap.new(bloomScreen)
bloomSprite:setScale(bloomScale,bloomScale)
bloomSprite:setColorTransform(0.96,0.96,0.96) -- this is the decay, smaller for faster decay
screenRender=Sprite.new()
screenRender:setScale(screenSizeX/width,screenSizeY/height)
screenRender:setBlendMode("add") |
Then in your main game enter_frame loop (I have one running constantly) add this just before the end:
local oo=(oddEven%2)+1
oddEven+=1
local oe=(oddEven%2)+1
bloomScreen:draw(bloomCapture[oe])
screenTexture[oe]:draw(bloomSprite)
screenTexture[oe]:draw(screenRender)
screenDraw[oe]:setVisible(true)
screenDraw[oo]:setVisible(false) |
Then just add all your game sprites to screenRender rather than stage. If you use sceneManager then add it like this rather than to stage...
screenRender:addChild(sceneManager) |
This is the result:
https://deluxepixel.com/players/bacteria/
Coder, video game industry veteran (since the '80s, ❤'s assembler), arrested - never convicted hacker (in the '90s), dad of five, he/him (if that even matters!).
https://deluxepixel.com
Comments
e.g
Likes: MoKaLux
https://deluxepixel.com
Likes: SinisterSoft
https://deluxepixel.com
Likes: SinisterSoft, MoKaLux
Instead of the scale down from the last drawn and then scale up and draw over the next draw, maybe a shader could be added to do that in one go - it would also save the bloom copy buffer.
The big advantage is though that it will work on all the platforms without having to make specific shaders - eg opengl shader language vs metal, etc...
https://deluxepixel.com
Likes: SinisterSoft
Fragmenter - animated loop machine and IKONOMIKON - the memory game
Likes: MoKaLux, keszegh
Fragmenter - animated loop machine and IKONOMIKON - the memory game
Fragmenter - animated loop machine and IKONOMIKON - the memory game
I played around with it but couldn't go very far. Let me post the code example and a shadertoy neon example: https://www.shadertoy.com/view/MsyGRm
I just wonder how he managed to get the shaders from shadertoy and put them into the shaders folder!?! Please somebody tell me
Likes: SinisterSoft
As an example, thats how you can get shader code:
APP_KEY you can get at https://www.shadertoy.com/myapps
For the API look here: https://www.shadertoy.com/howto
Likes: MoKaLux
https://deluxepixel.com
Fragmenter - animated loop machine and IKONOMIKON - the memory game
Steps you need to reproduce:
One RT for thresholded brightness texture, second is for blur, and the third one for a final result.
Likes: MoKaLux